Different Methods to Reverse a String in C++
Last Updated :
16 Feb, 2023
The reversing of a string is nothing but simply substituting the last element of a string to the 1st position of the string.
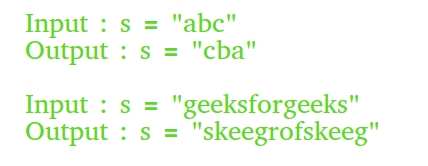
Different Methods to Reverse a String in C++ are:
- Making our own reverse function
- Using ‘inbuilt’ reverse function
- Using Constructor
- Using a temp file
1. Making a Custom Reverse Function For Swapping Characters
- Using a first to last approach ‘for’ loop
CPP
#include <bits/stdc++.h>
using namespace std;
void reverseStr(string& str)
{
int n = str.length();
for ( int i = 0; i < n / 2; i++)
swap(str[i], str[n - i - 1]);
}
int main()
{
string str = "geeksforgeeks" ;
reverseStr(str);
cout << str;
return 0;
}
|
Complexity Analysis:
Time Complexity: O(N)
Auxiliary Space: O(1)
- Using a first to last Approach with while loop
C++
#include <bits/stdc++.h>
using namespace std;
void reverseStr(string& str)
{
int len = str.length();
int n = len-1;
int i = 0;
while (i<=n){
swap(str[i],str[n]);
n = n-1;
i = i+1;
}
}
int main()
{
string str = "geeksforgeeks" ;
reverseStr(str);
cout << str;
return 0;
}
|
Complexity Analysis:
Time Complexity: O(N)
Auxiliary Space: O(1)
- Using a Last to First Approach ‘for‘ Loop
C++
#include <bits/stdc++.h>
using namespace std;
void reverse(string str)
{
for ( int i = str.length() - 1; i >= 0; i--)
cout << str[i];
}
int main( void )
{
string s = "GeeksforGeeks" ;
reverse(s);
return (0);
}
|
Complexity Analysis:
Time Complexity: O(N)
Auxiliary Space: O(1)
- Using a Last to First Approach ‘while’ Loop
C++
#include <bits/stdc++.h>
using namespace std;
void reverse(string str)
{
int len = str.length();
int n = len;
while (n--)
cout << str[n];
}
int main( void )
{
string s = "GeeksforGeeks" ;
reverse(s);
return (0);
}
|
Complexity Analysis:
Time Complexity: O(N)
Auxiliary Space: O(1)
1. Using recursion Function with two pointer approach
Recursion functions are used for iterating to different indexes of the string.
C++
#include <bits/stdc++.h>
using namespace std;
void reverseStr(string& str, int n, int i)
{
if (n<=i){ return ;}
swap(str[i],str[n]);
reverseStr(str,n-1,i+1);
}
int main()
{
string str = "geeksforgeeks" ;
reverseStr(str, str.length()-1, 0);
cout << str;
return 0;
}
|
Complexity Analysis:
Time Complexity: O(N)
Auxiliary Space: O(N)
2. Using one pointer approach in recursion
Below is the implementation of the code:
C++
#include <iostream>
using namespace std;
void getreverse(string &str, int i)
{
if (i > (str.length() - 1 - i))
{
return ;
}
swap(str[i], str[str.length() - i - 1]);
i++;
getreverse(str, i);
}
int main()
{
string name = "geeksforgeeks" ;
getreverse(name, 0);
cout << name << endl;
return 0;
}
|
Complexity Analysis:
Time Complexity: O(N)
Auxiliary Space: O(N)
3. Using the inbuilt “reverse” Function
There is a direct function in the “algorithm” header file for doing reverse that saves our time when programming.
// Reverses elements in [begin, end]
void reverse (BidirectionalIterator begin,
BidirectionalIterator end);
CPP
#include <bits/stdc++.h>
using namespace std;
int main()
{
string str = "geeksforgeeks" ;
reverse(str.begin(), str.end());
cout << str;
return 0;
}
|
Complexity Analysis:
Time Complexity: O(N)
Auxiliary Space: O(1)
4. Reverse a String Using the Constructor
Passing reverse iterators to the constructor returns us a reversed string.
CPP
#include <bits/stdc++.h>
using namespace std;
int main()
{
string str = "GeeksforGeeks" ;
string rev = string(str.rbegin(), str.rend());
cout << rev << endl;
return 0;
}
|
Complexity Analysis:
Time Complexity: O(N)
Auxiliary Space: O(1)
5. Using a Temporary String
CPP
#include <bits/stdc++.h>
using namespace std;
int main()
{
string str = "GeeksforGeeks" ;
int n = str.length();
string rev;
for ( int i = n - 1; i >= 0; i--)
rev.push_back(str[i]);
cout << rev << endl;
return 0;
}
|
Complexity Analysis:
Time Complexity: O(N)
Auxiliary Space: O(1)
How could we get the reverse of a const string?
To get the reverse of a const string we have to first declare a ‘const string’ in a user-defined function following which we have declared then use the following algorithm for the calling of the desired objects.
“const reverseConstString = function(string) { return string.split("").reverse().join("")”
Example:
C++
#include <bits/stdc++.h>
using namespace std;
char * reverseConstString( char const * str)
{
int n = strlen (str);
char * rev = new char [n + 1];
strcpy (rev, str);
for ( int i = 0, j = n - 1; i < j; i++, j--)
swap(rev[i], rev[j]);
return rev;
}
int main( void )
{
const char * s = "GeeksforGeeks" ;
printf ( "%s" , reverseConstString(s));
return (0);
}
|
Time Complexity: O(N)
Auxiliary Space: O(N)
Using Stack Data Structure
C++
#include <bits/stdc++.h>
using namespace std;
int main()
{
string s = "GeeksforGeeks" ;
stack< char > st;
for ( char x : s)
st.push(x);
while (!st.empty()) {
cout << st.top();
st.pop();
}
return 0;
}
|
Complexity Analysis:
Time Complexity: O(N)
Auxiliary Space: O(N)
This article is contributed by Priyam kakati, Ranju Kumari, Somesh Awasthi. If you like GeeksforGeeks and would like to contribute, you can also write an article at write.geeksforgeeks.org. See your article appearing on the GeeksforGeeks main page and help other Geeks. Please write comments if you find anything incorrect, or if you want to share more information about the topic discussed above.
Priyam kakati, Ranju Kumari, Somesh Awasthi
Please Login to comment...