ReactJS Tutorials
Last Updated :
16 Jan, 2023
React is a declarative, efficient, and flexible JavaScript library for building user interfaces. It is an open-source, component-based front-end library that is responsible only for the view layer of the application. ReactJS is not a framework, it is just a library developed by Facebook to solve some problems that we were facing earlier.
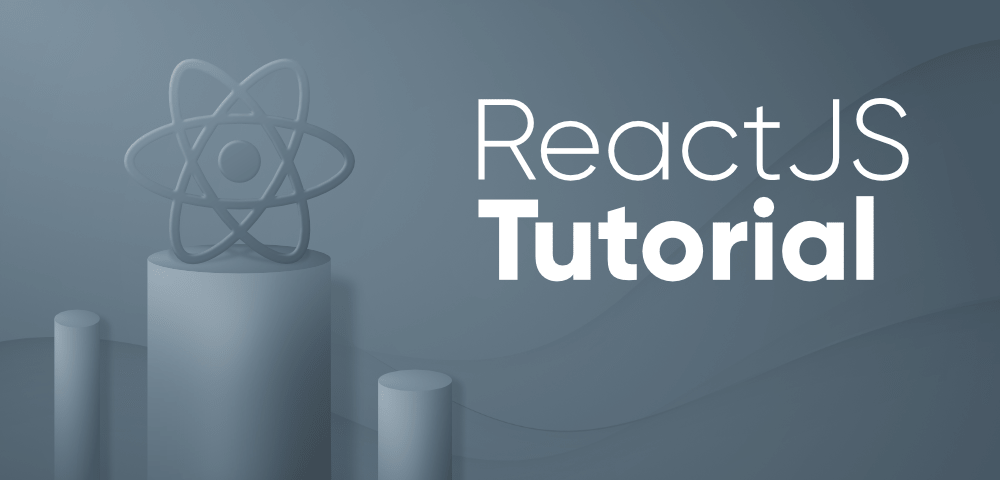
React is a declarative, efficient, and flexible JavaScript library for building user interfaces. It is a Model-View-Controller (MVC) architecture-based library that plays the role of “V” which means view. It designs simple views for each state in your application, and React will efficiently update and render just the right component when your data changes. The declarative view makes your code more predictable and easier to debug.
Prerequisite:
Before you start learning ReactJS, we assume that you have knowledge of HTML, CSS and JavaScript. There are few others skill sets that will help you to complete this tutorial at your best pace.
- Use JSX: JSX is faster than normal JavaScript as it performs optimizations while translating to regular JavaScript. It makes it easier for us to create templates.
- Virtual DOM: Virtual DOM exists which is like a lightweight copy of the actual DOM. So for every object that exists in the original DOM, there is an object for that in React Virtual DOM. It is exactly the same, but it does not have the power to directly change the layout of the document. Manipulating DOM is slow, but manipulating Virtual DOM is fast as nothing gets drawn on the screen.
- One-way Data Binding: One-way data binding gives you better view over your application.
- Component: A Component is one of the core building blocks of React. In other words, we can say that every application you will develop in React will be made up of pieces called components. Components make the task of building UIs much easier.
- Performance: ReactJS use JSX, which is faster compared to normal JavaScript and HTML. Virtual DOM is a less time taking procedure to update webpages content.
Example: This example is only to give you an idea of how JSX looks. In this whole tutorial, we will use JSX.
App.js
import React from "react";
import ReactDOM from "react-dom";
var name = "Learner";
var element = <h1>Hello, {name}.Welcome to GeeksforGeeks.</h1>;
ReactDOM.render(element, document.getElementById("root"));
Output:

- Composable: We can divide these codes and put them in custom components. Then we can utilize those components and integrate them into one place.
- Declarative: In react the DOM is declarative. We can make interactive UIs by changing the state of the component and React takes care of updating the DOM according to it.
- SEO Friendly: React affects the SEO by giving you a SPA (Single Page Application) which requires Javascript to show the content on the page which can be rendered and indexed.
- Community: React has a huge community because of it’s demand each company wants to work with React. Companies like Meta, Netflix, etc built on React.
- Easy to learn: HTML-like syntax of JSX make you comfortable with the codes of React, it only requires to need a basic knowledge of HTML, CSS, and JS fundamentals to start working with it.
Learn more about ReactJS:
ReactJS Complete References:
Your first React App:
A Project will always help you to be confident in your learning path, so we recommend you to follow the bellow mentioned articles after you clear your fundamentals of React by following our React Basics section.
Share your thoughts in the comments
Please Login to comment...